- Published on
Dynamic Import (Next.js)
- Authors
- Name
- Panuwat Boonrod
- @nilpanuwat
Follow the Thai version here.
Overview
The other day, I encountered a feature in Next.js that I tried out and found to be beneficial. I'd like to share it for those who may not be familiar yet, as it could be very useful for optimizing our app to run faster.
Normally, when we write Next.js and a component needs to toggle or show/hide on a particular page, we typically use state to determine when to display component B when the state changes. We might write it like this:
import Home from "../features/Home";
import Modal from "../features/Modal";
import { useState } from 'react'
export default function Index() {
const [open, setOpen] = useState(false)
const handleClick = () => {
setOpen(true);
};
return open ? <Modal /> : <Home onClick={handleClick} />;
}
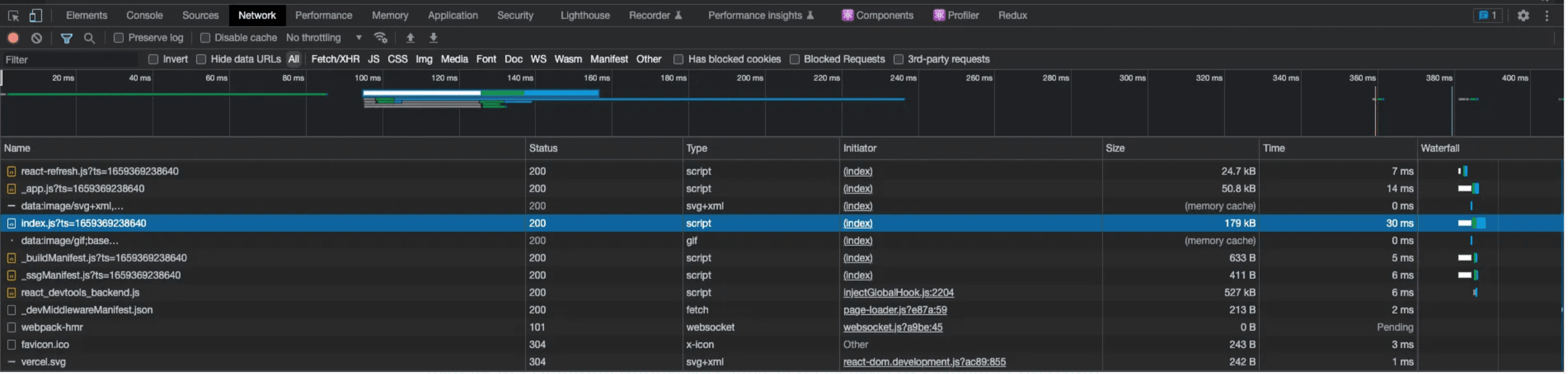
Size 179kb
To ensure that the Modal
component is loaded but not yet actively used, you can utilize Dynamic import in Next.js. This approach ensures that Modal
is not loaded until it is actually invoked, as indicated by the file size of the .js file and the increase in .js files when triggered for functionality.
After that...
import { useState } from 'react'
import dynamic from "next/dynamic"
const Home = dynamic(()) => import("../features/Home"));
const Modal = dynamic(()) => import("../features/Modal"));
export default function Index() {
const [open, setOpen] = useState(false)
const handleClick = () => {
setOpen(true);
};
return open ? <Modal /> : <Home onClick={handleClick} />;
}
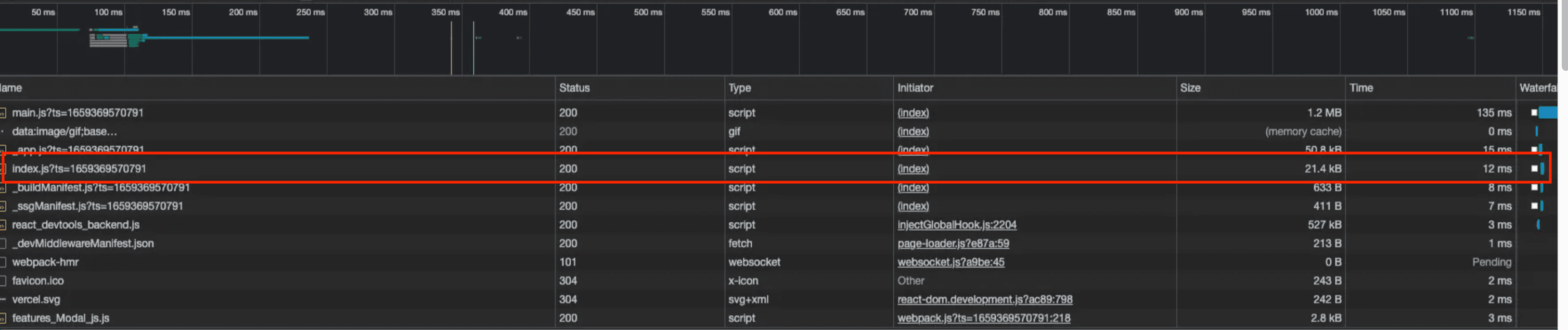
Size 21.4kb and modal.js was added after that component was triggered
Conclusion
Dynamic import helps with reducing the initial loading time of an application by allowing components or modules to be loaded only when they are needed.
Imagine if we have more than 10 components, or even many more. Dynamic import enables us to load these components asynchronously, meaning they are fetched from the server and initialized only when they are actually used in the application. This approach significantly improves the initial load performance of the app by deferring the loading of components that are not immediately required.
refs: https://nextjs.org/docs/pages/building-your-application/optimizing/lazy-loading